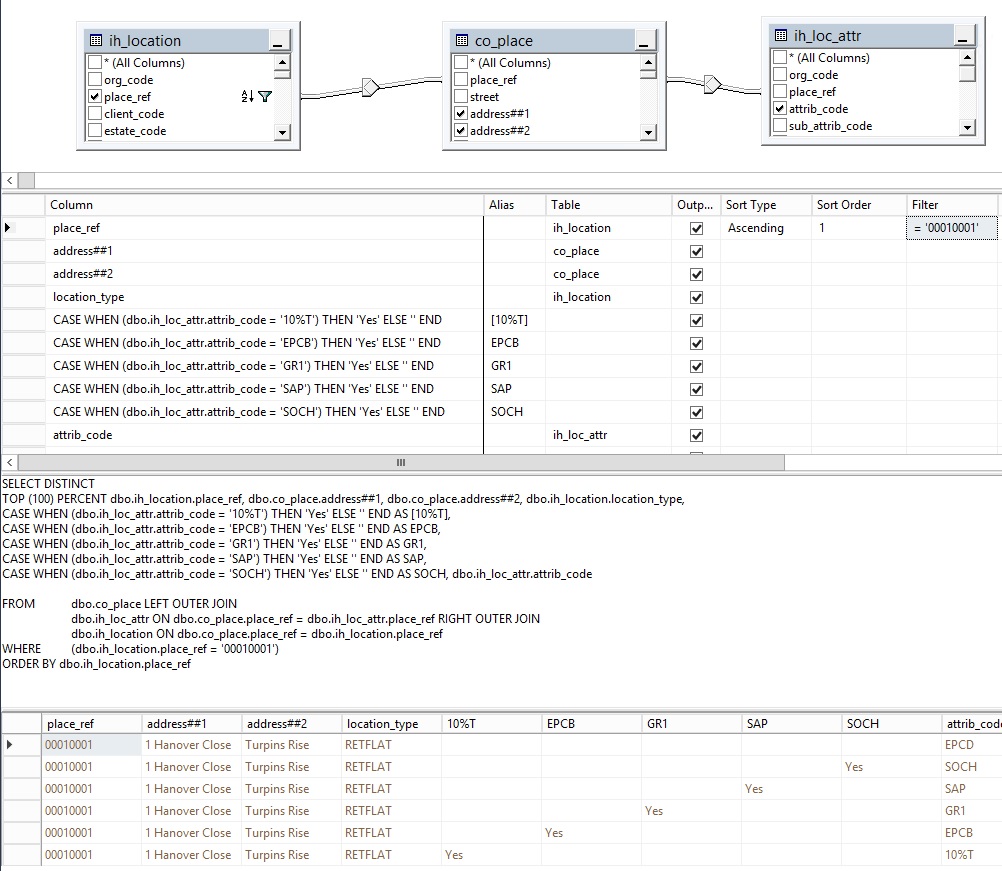
The case when sql statement in SQL is a powerful tool that allows you to add conditional logic to your queries. This function is similar to the IF-THEN-ELSE
logic found in many programming languages. With case when sql, you can perform complex filtering, data manipulation, and even error checking directly in your SQL queries. In this guide, we will explore how to use case when sql effectively, including syntax, practical examples, and best practices.
Understanding the Basics of CASE WHEN SQL
The case when sql statement allows you to create a conditional logic structure within SQL, enabling you to control the output based on certain conditions. The basic syntax for a case when sql statement in SQL is:
SELECT column1,
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
...
ELSE resultN
END as new_column_name
FROM table_name;
Here’s what each part of the syntax means:
CASE
: This keyword introduces the conditional logic block.WHEN
: Defines the condition to check.THEN
: Specifies the result if theWHEN
condition is true.ELSE
: (Optional) Provides an alternative result if none of theWHEN
conditions are met.END
: Closes theCASE
statement.
Why Use CASE WHEN SQL?
Using case when sql queries offers several advantages:
- Data Transformation: You can transform data within your query, such as categorizing data or converting numerical values into descriptive labels.
- Conditional Calculations: Perform calculations based on conditions without creating additional columns in the table.
- Enhanced Filtering: Create dynamic filters to retrieve specific data based on complex conditions.
- Error Handling: Manage unexpected or null values by providing a default outcome.
Practical Examples of CASE WHEN SQL
Let’s look at some practical examples to understand how case when sql can be used effectively in SQL queries.
Example 1: Categorizing Data
Suppose you have a table named Employees
with a column Salary
. You want to create a new column called SalaryRange
that categorizes employees based on their salary.
SELECT EmployeeID,
EmployeeName,
Salary,
CASE
WHEN Salary < 30000 THEN 'Low'
WHEN Salary BETWEEN 30000 AND 70000 THEN 'Medium'
WHEN Salary > 70000 THEN 'High'
ELSE 'Not Specified'
END as SalaryRange
FROM Employees;
In this example:
- Employees earning less than 30,000 are categorized as ‘Low’.
- Salaries between 30,000 and 70,000 are labeled as ‘Medium’.
- Salaries above 70,000 are considered ‘High’.
- If the salary data is missing, the result is ‘Not Specified’.
Example 2: Conditional Calculations
Imagine a scenario where you have sales data in a table called Sales
and you want to calculate a commission based on different criteria:
SELECT SalesID,
SalesAmount,
CASE
WHEN SalesAmount >= 10000 THEN SalesAmount * 0.1
WHEN SalesAmount >= 5000 THEN SalesAmount * 0.05
ELSE SalesAmount * 0.02
END as Commission
FROM Sales;
Here, the commission rate varies based on the SalesAmount
:
- Sales above 10,000 earn a 10% commission.
- Sales between 5,000 and 9,999 earn a 5% commission.
- Sales below 5,000 earn a 2% commission.
Example 3: Handling NULL Values
In SQL, handling NULL
values can be tricky. Let’s say you have a table named Orders
with a column Discount
. You want to replace NULL
values in Discount
with 0:
SELECT OrderID,
OrderAmount,
CASE
WHEN Discount IS NULL THEN 0
ELSE Discount
END as FinalDiscount
FROM Orders;
This query checks if the Discount
is NULL
. If it is, it returns 0; otherwise, it returns the actual Discount
.
Advanced Use Cases of CASE WHEN
While the above examples cover basic scenarios, case when sql can also handle more advanced SQL functionalities.
Example 4: Nested CASE Statements
You can nest case when sql statements within each other to manage more complex logic. For example:
SELECT ProductID,
ProductName,
Price,
CASE
WHEN Price < 100 THEN 'Budget'
WHEN Price BETWEEN 100 AND 500 THEN
CASE
WHEN Category = 'Electronics' THEN 'Affordable Electronics'
ELSE 'Affordable Non-Electronics'
END
ELSE 'Premium'
END as PriceCategory
FROM Products;
In this case, the PriceCategory
column depends not only on the Price
but also on the Category
of the product.
Example 5: Using CASE WHEN with Aggregate Functions
Case when sql can be used alongside aggregate functions to provide grouped calculations. Suppose you want to calculate the total sales amount per product category but only for specific conditions:
SELECT Category,
SUM(CASE WHEN SalesDate >= '2024-01-01' THEN SalesAmount ELSE 0 END) as TotalSalesYTD
FROM Sales
GROUP BY Category;
This query calculates the total sales amount for each category but only includes sales from the year 2024 onwards.
Best Practices for Using CASE WHEN SQL
While CASE WHEN
is a versatile tool, it’s essential to follow best practices to ensure optimal performance and maintainable code:
- Keep It Simple: Avoid overly complex
CASE
statements. If the logic is too complicated, consider breaking it into smaller, manageable pieces or using other SQL functions. - Watch for Performance: Complex or multiple
CASE
statements can slow down query performance, especially on large datasets. Use indexing and query optimization techniques as needed. - Use ELSE Sparingly: The
ELSE
clause is optional but can be helpful for handling unexpected cases orNULL
values. However, ensure it doesn’t mask potential data quality issues. - Test Extensively: Always test your queries with various data inputs to ensure the
CASE WHEN
logic works as intended and produces the correct results. - Document Your Queries: Add comments or documentation to your SQL scripts to explain the logic behind your
CASE
statements, making it easier for others (or your future self) to understand.
Conclusion
The case when sql statement is an invaluable feature in SQL that can significantly enhance your data analysis capabilities. Whether you need to categorize data, perform conditional calculations, handle NULL
values, or implement complex logic within your queries, case when sql provides a flexible solution. By mastering its use and following best practices, you can write more powerful and efficient SQL queries, making your data work harder for you.
Explore case when sql in your SQL environment, experiment with its capabilities, and you’ll find it an indispensable tool in your SQL toolkit.